Difference between session.save, session.saveOrUpdate and session.persist? Session.save: Save does an insert and will fail if the primary key is already persistent. Session.saveOrUpdate: saveOrUpdate does a select first to determine if it needs to do an insert or an update.Insert data if primary key not exist otherwise update data. You should apply the differnce between save and saveOrUpdate method in your code to get the best performance: The save method returns the identifier generated by the database. On the other hand, saveOrUpdate can do INSERT or UPDATE depending upon whether object exists in database or not.
- In this video you will learn about Methods like Save-persist-saveOrUpdate Using a demo projectBelow is the GitHub link to download source:https://githu.
- So saveOrUpdate method calls save method if there is no record in database, and it calls update method if there is a record in database. Related Posts: getTransaction,beginTransaction,getIdentifier in Hibernate; CRUD operations using Hibernate+ Maven+ Oracle+ XML mapping.
1. Overview
In this tutorial, we'll discuss what cascading is in JPA/Hibernate. Then we'll cover the various cascade types that are available, along with their semantics.
Further reading:
Introduction to Spring Data JPA
Mapping Entity Class Names to SQL Table Names with JPA
2. What Is Cascading?
Entity relationships often depend on the existence of another entity, for example the Person–Address relationship. Without the Person, the Address entity doesn't have any meaning of its own. When we delete the Person entity, our Address entity should also get deleted.
Cascading is the way to achieve this. When we perform some action on the target entity, the same action will be applied to the associated entity.
2.1. JPA Cascade Type
All JPA-specific cascade operations are represented by the javax.persistence.CascadeType enum containing entries:
- ALL
- PERSIST
- MERGE
- REMOVE
- REFRESH
- DETACH
2.2. Hibernate Cascade Type
Hibernate supports three additional Cascade Types along with those specified by JPA. These Hibernate-specific Cascade Types are available in org.hibernate.annotations.CascadeType:
- REPLICATE
- SAVE_UPDATE
- LOCK
3. Difference Between the Cascade Types
3.1. CascadeType.ALL
CascadeType.ALLpropagates all operations — including Hibernate-specific ones — from a parent to a child entity.
Let's see it in an example:
Note that in OneToMany associations, we've mentioned cascade type in the annotation.
Now let's see the associated entity Address:
3.2. CascadeType.PERSIST
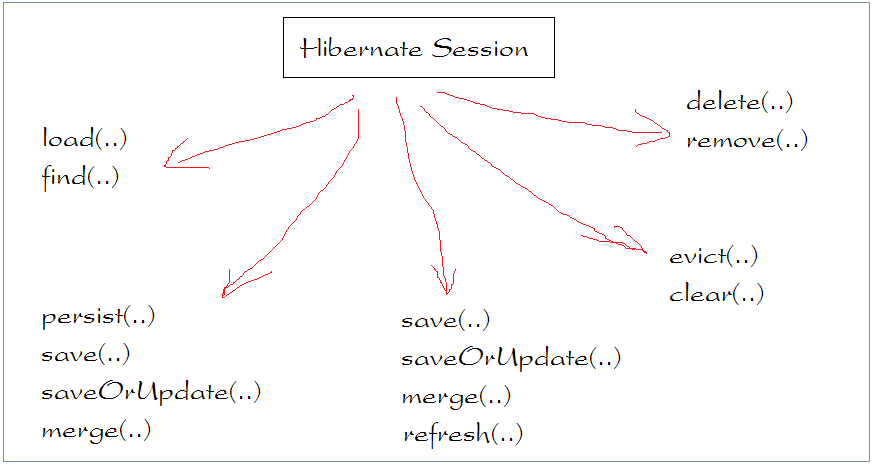
The persist operation makes a transient instance persistent. Cascade Type PERSIST propagates the persist operation from a parent to a child entity. When we save the person entity, the address entity will also get saved.
Let's see the test case for a persist operation:
When we run the above test case, we'll see the following SQL:
3.3. CascadeType.MERGE
The merge operation copies the state of the given object onto the persistent object with the same identifier. CascadeType.MERGE propagates the merge operation from a parent to a child entity.
Let's test the merge operation:
When we run the test case, the merge operation generates the following SQL:
Here, we can see that the merge operation first loads both address and person entities and then updates both as a result of CascadeType.MERGE.
3.4. CascadeType.REMOVE
As the name suggests, the remove operation removes the row corresponding to the entity from the database and also from the persistent context.
CascadeType.REMOVE propagates the remove operation from parent to child entity.Similar to JPA's CascadeType.REMOVE, we have CascadeType.DELETE, which is specific to Hibernate. There is no difference between the two.
Now it's time to test CascadeType.Remove:
When we run the test case, we'll see the following SQL:
The address associated with the person also got removed as a result of CascadeType.REMOVE.
3.5. CascadeType.DETACH
The detach operation removes the entity from the persistent context. When we use CascadeType.DETACH, the child entity will also get removed from the persistent context.
Let's see it in action:
Here, we can see that after detaching person, neither person nor address exists in the persistent context.
3.6. CascadeType.LOCK
Unintuitively, CascadeType.LOCK reattaches the entity and its associated child entity with the persistent context again.
Let's see the test case to understand CascadeType.LOCK:
As we can see, when using CascadeType.LOCK, we attached the entity person and its associated address back to the persistent context.
3.7. CascadeType.REFRESH
Refresh operations reread the value of a given instance from the database. In some cases, we may change an instance after persisting in the database, but later we need to undo those changes.
In that kind of scenario, this may be useful. When we use this operation with Cascade Type REFRESH, the child entity also gets reloaded from the database whenever the parent entity is refreshed.
For better understanding, let's see a test case for CascadeType.REFRESH:
Here, we made some changes in the saved entities person and address. When we refresh the person entity, the address also gets refreshed.
3.8. CascadeType.REPLICATE
The replicate operation is used when we have more than one data source and we want the data in sync. With CascadeType.REPLICATE, a sync operation also propagates to child entities whenever performed on the parent entity.
Now let's test CascadeType.REPLICATE:
Because of CascadeType.REPLICATE, when we replicate the person entity, its associated address also gets replicated with the identifier we set.
3.9. CascadeType.SAVE_UPDATE
CascadeType.SAVE_UPDATE propagates the same operation to the associated child entity. It's useful when we use Hibernate-specific operations like save, update and saveOrUpdate.
Let's see CascadeType.SAVE_UPDATE in action:
Because of CascadeType.SAVE_UPDATE, when we run the above test case, we can see that the person and address both got saved.
Here's the resulting SQL:
Update Vs Saveorupdate Hibernate
4. Conclusion
In this article, we discussed cascading and the different cascade type options available in JPA and Hibernate.
The source code for the article is available on GitHub.
hibernate works only with persistent entities and persistent entities are classes which are attached to any hibernate session. Please note that creating an instance of a class, you mapped with a hibernate annotations, does not automatically persist the object to the database. It must be save explicitly after attaching it to a valid hibernate session.
In this tutorial, learn to use hibernate save() and saveOrUpdate() methods under different usecases.
1. Hibernate save() method
In hibernate, we generally use one of below two versions of save() method:
Both save() methods take a transient object reference (which must not be null) as an argument. Second method takes an extra parameter ‘entityName‘ which is useful in case you have mapped multiple entities to a Java class. Here you can specify which entity you are saving using save() method.
A simple example to demo hibernate save() method.
Now let’s save this hibernate entity.
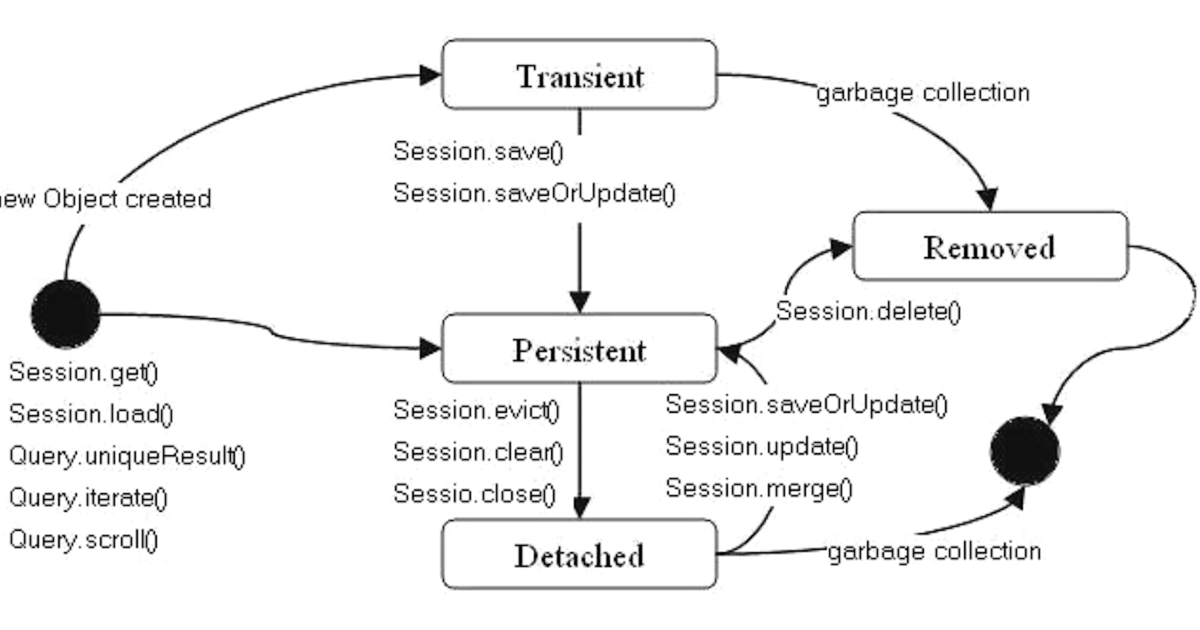
Program Output.
1.1. Calling save() method on persistent entity
We got our Employee
entity saved. So easy. But in reality, it is not so simple usecase. There you may need to update again employee entity and then save again in another session. Should you call save()
method again? Let’s check out.
Program Output.
Here hibernate tried to insert the entity again. Though it was failed due to primary key check, but check may not be there for other entities and you may end up with duplicate rows.
Note: While second save()
method causes duplicate row in different sessions, BUT in same session they will work correct.
Look at below example.
Program Output.
It’s confusing. Right? Let’s make it simple. And rule is below:
save()
method on a persistent entity (entity associated with any hibernate session). Any changes done to persistent entity is automatically saved.1.2. Changing state of persistent entity
Any change to persistent entity is saved automatically. Let’s understand this concept in simple example.
Program Output.
In above example, we made the ‘emp
‘ object persistent using first save()
method. Afterward when we updated the last name to ‘temp‘, an update query was executed as expected. This we verified in returned data as well. This is the correct way to work with hibernate persistent entities.
Difference Between Update And Saveorupdate In Hibernate 2019
2. Hibernate saveOrUpdate() method
In discussion of save()
method, we forgot about case where we had to save persistent entity in another session and that got resulted in duplicate key error. That is also a valid scenario.
To handle such cases, you must use saveOrUpdate()
method. Strictly speaking, you should use saveOrUpdate() with even non-persistent entities. Personally, I do not see any harm in doing so. Though, It may make you a little bit careless. So be cautious.
2.1. Hibernate saveOrUpdate() example
Let’s see how saveOrUpdate()
method can be used along with entity persisted with save()
method.
Program Output.
Now we are able to save the entity as well as update the entity as well using saveOrUpdate()
method.
Please remember that if you have used saveOrUpdate()
method in place of save()
method above, then also result would have been same. saveOrUpdate()
can be used with persistent as well as non-persistent entities both. Persistent entities will get updated, and transient entities will be inserted into database.
3. Suggestion For production code – best practices
It wouldn’t be advisable to try to use above code in production code. Ideally, what you would do is pass VO object to DAO layer, load the entity from the session and update the entity with by copying VO data onto it. This means that the updates take place on a persistent object, and we don’t actually have to call Session.save()
or Session.saveOrUpdate()
at all.
Once an object is in a persistent state, Hibernate manages updates to the database itself as you change the fields and properties of the object. It’s big relief.
Difference Between Save And Saveorupdate In Hibernate
4. Summary
- Save() method stores an object into the database. It will Persist the given transient instance, first assigning a generated identifier. It returns the id of the entity created.
- SaveOrUpdate() calls either save() or update() on the basis of identifier exists or not. e.g if identifier does not exist, save() will be called or else update() will be called.
- Probably you will get very few chances to actually call save() or saveOrUpdate() methods, as hibernate manages all changes done in persistent objects.
Let me know is something is not clear or needs more explanation related to hibernate save() and saveOrUpdate() methods.
Difference Between Update And Saveorupdate In Hibernate Windows 10
Happy Learning !!